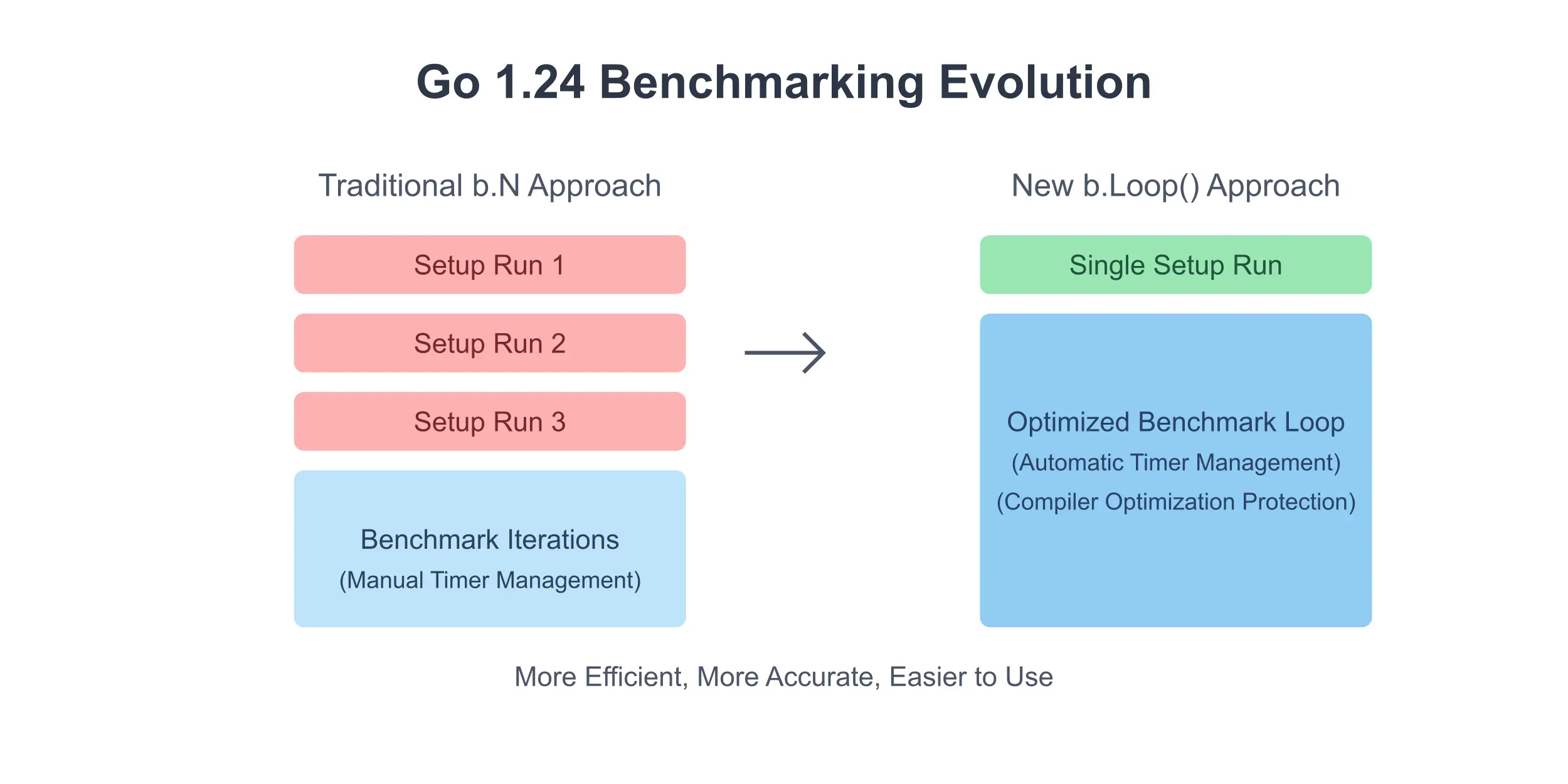
Go 1.24's New Benchmark Function; a better way to benchmark
Go 1.24 introduces a significant improvement to how we write benchmarks with the new testing.B.Loop
method. This change makes benchmarking both faster and less error-prone, addressing some long-standing issues with the traditional benchmarking approach.
The Traditional Way
Historically, Go developers wrote benchmarks using b.N
like this:
func BenchmarkMyFunction(b *testing.B) {
// Setup code might run multiple times
expensiveSetup()
for range b.N {
MyFunction()
}
}
While this pattern works, it has several issues:
- Setup code runs multiple times as the framework calibrates
b.N
- Setup timing needs manual management with
b.ResetTimer()
- The compiler might optimize away operations if results aren’t used
- The pattern can be error-prone for newcomers
Enter B.Loop: A Better Solution
The new B.Loop
method provides a cleaner, more efficient approach:
func BenchmarkMyFunction(b *testing.B) {
// Setup code runs exactly once
expensiveSetup()
for b.Loop() {
MyFunction()
}
// After Loop returns false, b.N contains
// the total number of iterations that ran
}
Key Advantages
1. Automatic Timer Management
B.Loop
handles the benchmark timer automatically:
- It resets the timer on first call, so setup code isn’t counted
- When returning false, it stops the timer so cleanup isn’t counted
- No need to manually call
b.ResetTimer()
for setup code
2. Compiler Optimization Protection
One of the most significant improvements is how B.Loop
handles compiler optimizations:
- The compiler never optimizes away function calls within a
for b.Loop() { ... }
loop - This only applies to calls directly between the loop’s curly braces
- Functions called by the loop body are still optimized normally
- This prevents the compiler from eliminating benchmark code it thinks is unused
3. Single Execution Guarantee
With B.Loop
, the benchmark function runs exactly once per measurement:
- Setup and cleanup code execute only once
- More efficient for benchmarks with expensive initialization
- More accurate timing of the actual operations being measured
4. Access to Final Iteration Count
After B.Loop
returns false, b.N
contains the total number of iterations that ran. This makes it easy to compute other average metrics based on the total number of operations performed.
Migration Tips
To migrate existing benchmarks:
-
Replace the traditional loop:
for i := 0; i < b.N; i++ {
or
for range b.N {
with:
for b.Loop() {
-
Remove any
b.ResetTimer()
calls for setup code -B.Loop
handles this automatically -
Move expensive setup outside the loop - it will only run once now
Conclusion
The new testing.B.Loop
method in Go 1.24 represents a significant improvement in how we write benchmarks. It’s not just syntactic sugar - it provides real benefits in terms of:
- More accurate measurements by properly handling setup code
- Protection against unwanted compiler optimizations
- Cleaner, more intuitive benchmark code
- Better support for benchmarks with expensive setup
B.Loop
should be your go-to choice for benchmark tests going forward. The automatic timer management and optimization guarantees make it both easier to use and more reliable than the traditional b.N
approach.
Happy coding!