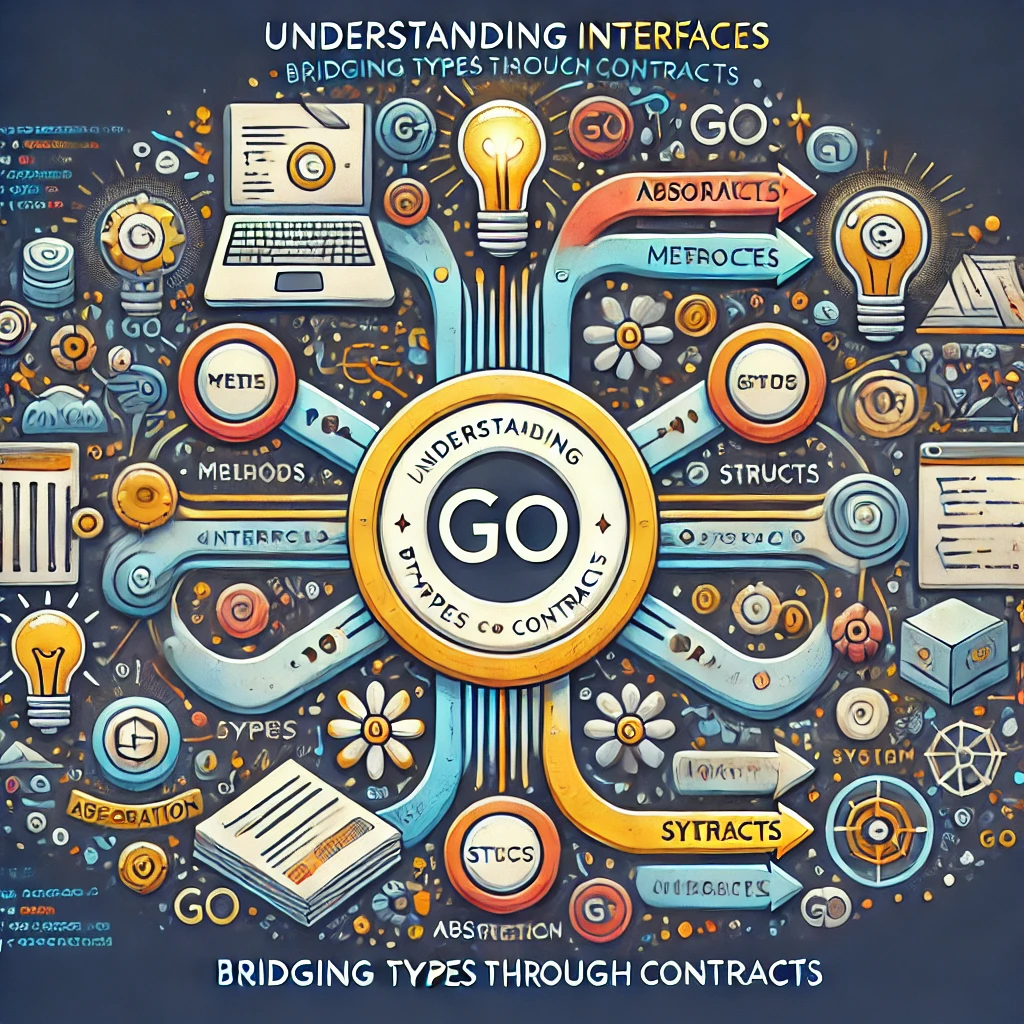
Explaining Go Interfaces | What They Are and Why They Matter
In the world of software development, creating flexible, scalable systems is vital.
In Go, one of the most valuable tools for achieving this is the interface. But what are Go interfaces, and why do they matter?
In this guide, we’ll simplify the concept of Go interfaces, explain their real-world applications, and discuss their benefits and challenges—without delving into technical code examples. This ensures the content is accessible to everyone, whether you’re an experienced developer or just starting out.
If you’re exploring Go programming, interfaces are only part of the story. You can expand your knowledge by exploring gRPC with Go for building high-performance APIs, mastering The Art of CLI Development in Go, or sharpening your skills with The Ultimate Guide to Debugging with Go. These resources can help deepen your understanding of Go and its many capabilities.
What Are Interfaces in Go?
At its core, an interface in Go is like a contract. It defines the actions or behaviors that a component should perform without specifying how it should perform them. For example, if you were designing a car, the interface might specify that the car needs to be able to start, stop, and accelerate, but it wouldn’t dictate whether the car runs on gasoline, electricity, or solar power. This level of abstraction allows developers to focus on behavior rather than implementation.
package main
import "fmt"
// Vehicle interface defines the behaviors any type of vehicle should have
type Vehicle interface {
Start()
Stop()
Accelerate()
}
// GasCar struct represents a gasoline-powered car
type GasCar struct {
Name string
}
// Start implements the Vehicle interface for GasCar
func (g GasCar) Start() {
fmt.Printf("%s is starting with a roar of the engine!\n", g.Name)
}
// Stop implements the Vehicle interface for GasCar
func (g GasCar) Stop() {
fmt.Printf("%s is stopping and turning off the engine.\n", g.Name)
}
// Accelerate implements the Vehicle interface for GasCar
func (g GasCar) Accelerate() {
fmt.Printf("%s is accelerating with gasoline power!\n", g.Name)
}
// ElectricCar struct represents an electric car
type ElectricCar struct {
Name string
}
// Start implements the Vehicle interface for ElectricCar
func (e ElectricCar) Start() {
fmt.Printf("%s is starting silently with electric power.\n", e.Name)
}
// Stop implements the Vehicle interface for ElectricCar
func (e ElectricCar) Stop() {
fmt.Printf("%s is stopping and conserving battery power.\n", e.Name)
}
// Accelerate implements the Vehicle interface for ElectricCar
func (e ElectricCar) Accelerate() {
fmt.Printf("%s is accelerating smoothly with electric motors!\n", e.Name)
}
func main() {
// Create instances of GasCar and ElectricCar
gasCar := GasCar{Name: "Gasoline Car"}
electricCar := ElectricCar{Name: "Electric Car"}
// A slice of Vehicle, demonstrating interface abstraction
vehicles := []Vehicle{gasCar, electricCar}
// Iterate through and perform actions on each vehicle
for _, vehicle := range vehicles {
vehicle.Start()
vehicle.Accelerate()
vehicle.Stop()
}
}
Why Are Interfaces Essential in Go?
Interfaces are critical for creating systems where individual components can evolve independently. For example, if you’re designing an application that processes data, an interface allows you to switch between different types of storage, such as a local file, a database, or a cloud service, without having to overhaul your entire system.
What Makes Go Interfaces Different?
One unique feature of Go is that interfaces don’t require explicit declarations. In other programming languages, you often have to declare that a class or object implements an interface. In Go, as long as a component fulfills the required behaviors, it automatically satisfies the interface. This implicit satisfaction keeps Go programs simple and uncluttered.
In the previous example, you’ll notice we do not need to say that GasCar
is a car, its implicit.
Practical Applications of Go Interfaces
1. Building Modular and Adaptable Systems
One of the biggest advantages of interfaces is the ability to design modular systems. For example, interfaces allow you to write functions or systems that don’t depend on specific implementations. This makes it easy to swap out one part of the system for another without disrupting the rest.
2. Enabling Collaboration Between Teams
In larger projects, teams often work on separate parts of the system. Interfaces act as a shared language between teams, ensuring that each component adheres to the expected behaviors. This promotes seamless integration and reduces misunderstandings during development.
3. Examples from the Go Standard Library
Go’s standard library is a masterclass in using interfaces effectively. It includes examples where interfaces unify behaviors across various tools. For instance, the library defines interfaces for reading and writing data, enabling developers to handle everything from file I/O to network communication without rewriting their code for each use case.
Benefits and Challenges of Go Interfaces
The Benefits of Interfaces
- Improved Flexibility: Interfaces allow you to write code that works with different implementations, making your systems more adaptable to change.
- Simplified Testing: When testing, you can replace real components with mock implementations, making it easier to isolate and debug specific parts of your application.
- Reusability: Components that adhere to interfaces can often be reused across different projects or systems, saving you time and effort.
The Challenges of Interfaces
- Overuse Can Complicate Systems: While interfaces are powerful, using them unnecessarily can make a system overly complex.
- Understanding Implicit Satisfaction: Developers new to Go might find the lack of explicit declarations confusing at first.
- Striking the Right Balance: Creating interfaces that are broad enough to be flexible but specific enough to be useful is not always easy.
- How to Overcome Challenges Use interfaces only when there’s a clear need for abstraction. Favor smaller, purpose-driven interfaces over large, generic ones. Here is an example from the standard library for a reader:
type Reader interface {
Read(p []byte) (n int, err error)
}
Continuously review and refine your interfaces as the project evolves to ensure they remain relevant and effective.
Tips for Using Go Interfaces Effectively
1. Identify Clear Use Cases
Interfaces are most effective when there are multiple ways to perform an action or when a system’s implementation is likely to change. For example, an interface might be useful in defining how a system processes payments, as you might need to support various payment providers in the future.
2. Focus on Behavior, Not Implementation
The key to designing effective interfaces is to concentrate on what needs to be done, not how it’s done. This approach allows different implementations to coexist and makes it easier to adapt your system as requirements change.
3. Learn from Practical Examples
The Go standard library offers plenty of real-world examples of interfaces in action. By examining how the library uses interfaces for common tasks, you can gain insights into designing your own.
4. Avoid Overengineering
Start with specific implementations and introduce interfaces only when you see a clear benefit. This approach prevents unnecessary complexity and ensures that your interfaces remain practical and focused.
FAQs About Go Interfaces
1. What is the purpose of interfaces in Go?
Interfaces define expected behaviors, enabling developers to create flexible, modular systems that focus on actions rather than specific implementations.
2. How do interfaces improve collaboration in large projects?
By serving as a contract between different components, interfaces ensure smooth communication and integration between teams or system parts.
3. Are Go interfaces easy to use?
Yes. While the concept of implicit satisfaction may take some time to understand, it simplifies code by reducing the need for explicit declarations.
4. Can interfaces make testing easier?
Absolutely. By replacing real components with mock implementations, interfaces allow developers to test specific parts of their systems in isolation.
5. What are the best practices for designing interfaces in Go?
- Keep interfaces small and focused on specific behaviors.
- Introduce interfaces only when needed.
- Regularly review and refine interfaces to ensure they align with project goals.
Conclusion
Go interfaces are an essential tool for creating flexible, maintainable, and scalable systems. By focusing on behavior rather than implementation, they make it easier to design systems that adapt to changing requirements while remaining efficient and effective.
If you’re just beginning your journey with Go or looking to refine your skills, mastering interfaces is a great step. But don’t stop there - explore related topics like gRPC with Go, The Art of CLI Development in Go, and The Ultimate Guide to Debugging with Go. These resources will deepen your understanding and help you tackle more advanced challenges in Go development.
Go is a language that rewards clarity and simplicity, and interfaces are a perfect example of this philosophy in action. Embrace them, and you’ll find your systems easier to build, maintain, and evolve.